월루를 꿈꾸는 대학생
[Spring Boot] 간단한 RestApi 만들기 본문
728x90
먼저 간단하게 스프링 부트 프로젝트 생성
데이터 모델이 될 userProfile을 생성
public class UserProfile {
private String id;
private String name;
private String phone;
private String address;
public UserProfile(String id, String name, String phone, String address) {
this.id = id;
this.name = name;
this.phone = phone;
this.address = address;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
이제 컨트롤러에서 위의 객체를 만들고 request response를 보내주는 작업시작
먼저 간단하게 반환할 객체를 맵형태로 만듦
id를 키로 user객체를 밸류로 세팅
@PostConstruct를 통해서 프레임워크가 동작시 해당 userMap을 초기화
private Map<String, UserProfile> userMap;
// 스프링 프레임워크가 컨트롤러 인스턴스 생성시 초기화 해줌
@PostConstruct
private void init() {
userMap = new HashMap<String, UserProfile>();
userMap.put("1", new UserProfile("1", "홍길동", "111-1111", "서울시 강남구 대치동1"));
userMap.put("2", new UserProfile("2", "홍길동", "111-1112", "서울시 강남구 대치동2"));
userMap.put("3", new UserProfile("3", "홍길동", "111-1113", "서울시 강남구 대치동3"));
System.out.println(userMap);
}
GetMapping
// @PathVariable("id") 요 아이디가 path에 있는 id로 파라미터로 가져오도록 함
@GetMapping("/user/{id}")
public UserProfile getUserProfile(@PathVariable("id") String id) {
// UserProfile 객체 리턴하면 자동으로 JSON형태로 만들어줌
return userMap.get(id);
}
@GetMapping("user/all")
public List<UserProfile> getUserProfileList(){
//맵에 있는 리스트를 array value로 리턴
return new ArrayList<UserProfile>(userMap.values());
}
PutMapping
@PutMapping("/user{id}")
// http 형태의 파라미터로 값 전달
public void putUserProfile(@PathVariable("id") String id, @RequestParam("name") String name, @RequestParam("phone") String phone, @RequestParam("address") String address) {
// 데이터 생성시 패스로 아이디 받고 , 파라미터로 나머지 값을 받네
UserProfile userProfile = new UserProfile(id, name, phone, address);
//위에서 만든 객체 추가
userMap.put(id, userProfile);
}
데이터 수정 post
@PostMapping("/user/{id}")
public void postUserProfile(@PathVariable("id") String id, @RequestParam("name") String name, @RequestParam("phone") String phone, @RequestParam("address") String address) {
//얘는 수정용임
UserProfile userProfile = userMap.get(id);
userProfile.setName(name);
userProfile.setPhone(phone);
userProfile.setAddress(address);
}
삭제
@DeleteMapping("/user/{id}")
public void deleteUserProfile(@PathVariable("id") String id){
userMap.remove(id);
}
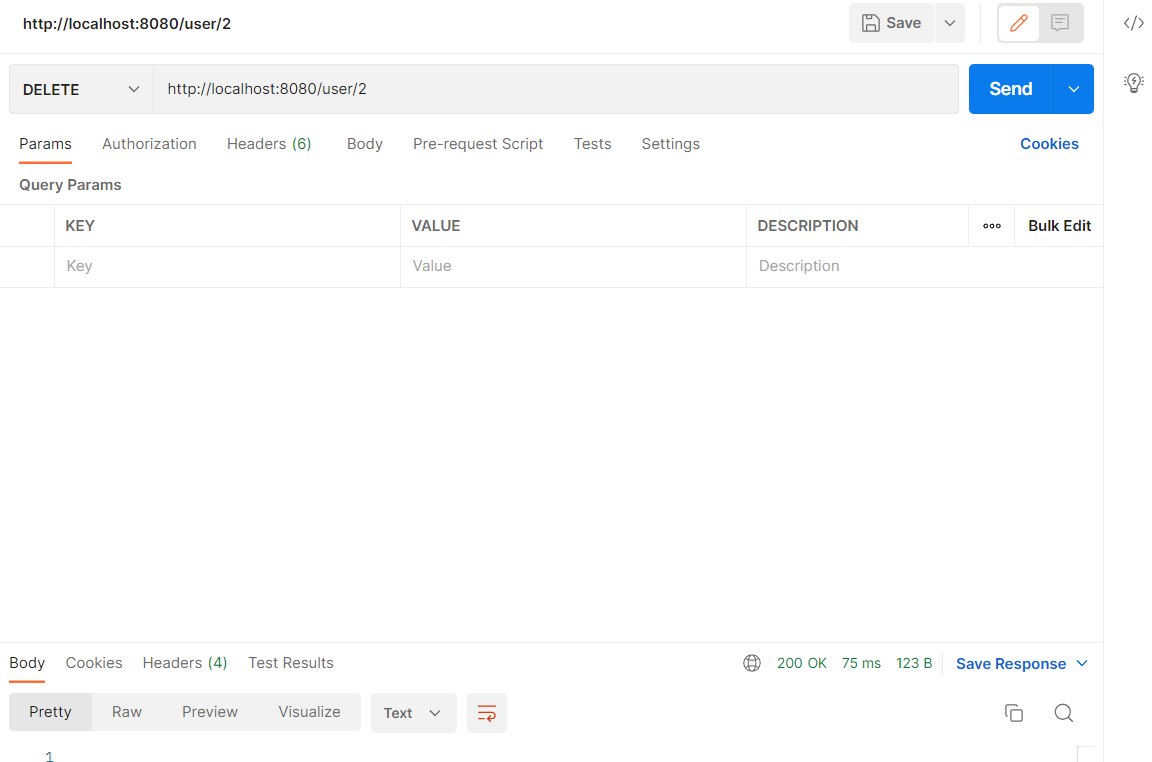
정리용 소스 코드
https://github.com/suhyun96/SpringBoot/tree/main/RestAPI
GitHub - suhyun96/SpringBoot: 스프링 부트 공부한 내용 정리용
스프링 부트 공부한 내용 정리용. Contribute to suhyun96/SpringBoot development by creating an account on GitHub.
github.com
출처
728x90
'Programing > Spring Boot' 카테고리의 다른 글
[GIT] .gitignore 관리 (0) | 2022.12.23 |
---|---|
[Spring Boot] Rest API & MySQL & MyBatis 연동 (0) | 2022.12.23 |
[Spring Boot] 리포지토리 (0) | 2022.12.23 |
[Spring Boot] 데이터 베이스 세팅 JPA (0) | 2022.12.15 |
[Spring Boot] 기본 세팅 및 기초 정리 (0) | 2022.12.15 |